Android Uninstall Tracking
App uninstall tracking through Firebase Messaging is supported by Airbridge Android SDK
v2.6.0
and later.
Introduction to Uninstall Tracking
Airbridge sends out daily silent push notifications using Google's FCM (Firebase Cloud Messaging) service to devices that have been registered within the last 6 months.
Uninstall data can be seen through Actuals Report, Raw Data Export, AWS S3 Export, and GCP Export.
Firebase Project Setup
Firebase Console
Go to the Firebase Console to create a project for your application.
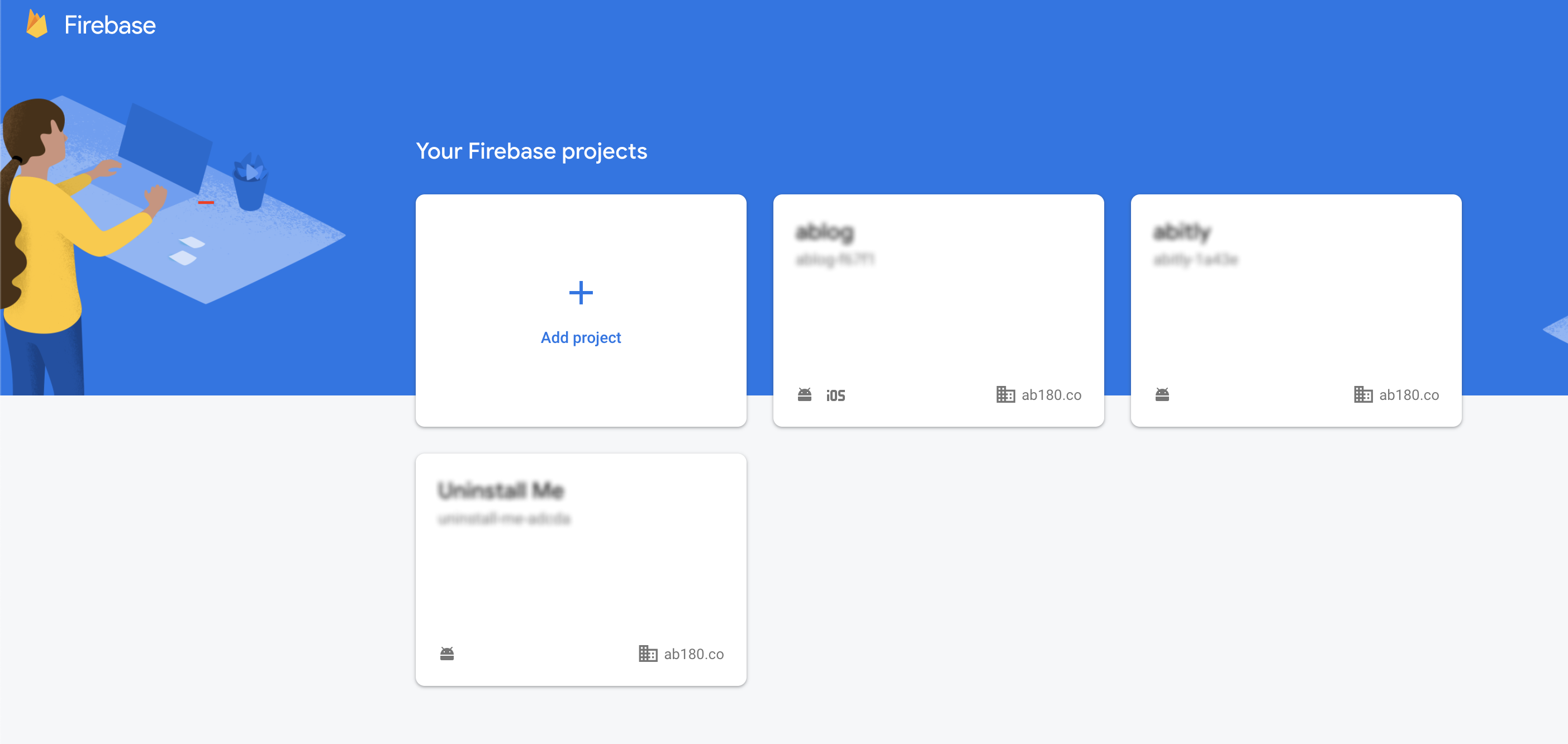
Server Key for Firebase Cloud Messaging
Go to "Project settings" by clicking on the gear icon on the top-left of the project overview page. Select the "Cloud Messaging" tab and find your server key as shown below.
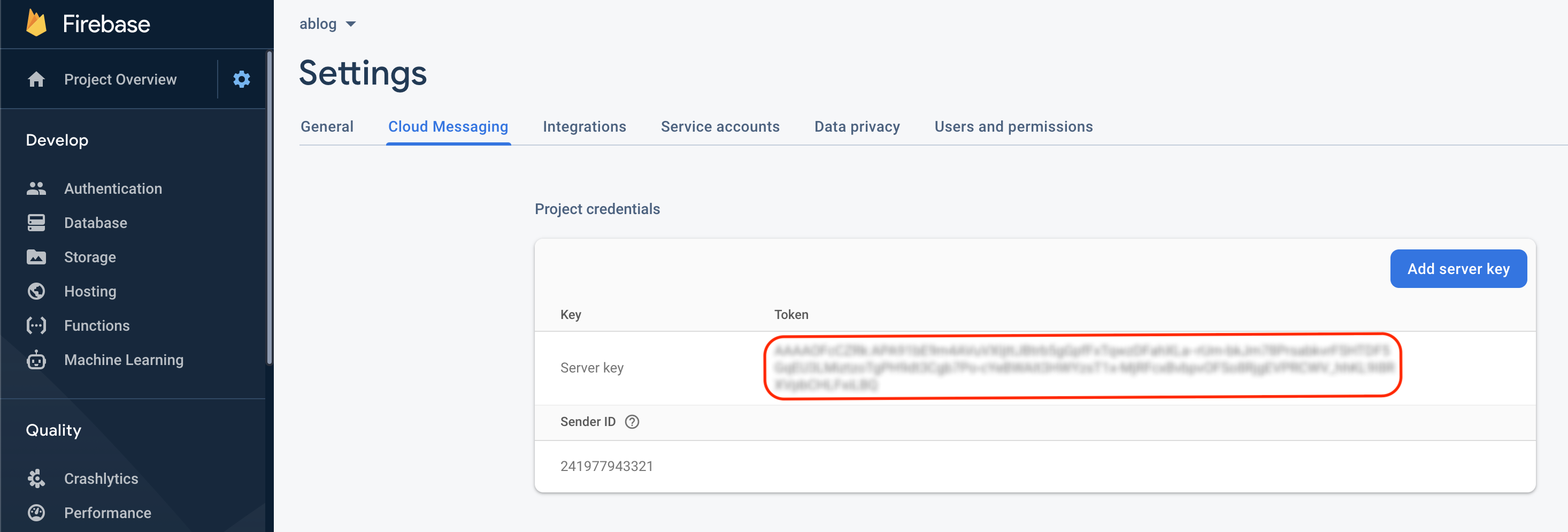
Firebase Cloud Messaging Setup
Please refer to this documentation to complete the basic Firebase Cloud Messaging setup for your application.
Firebase Push Token
Add the following code to AndroidManifest.xml
.
<service
android:name="${packageName}.MyFirebaseMessagingService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
Send the Firebase push token to Airbridge using the Airbridge SDK.
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
}
@Override
public void onNewToken(String token) {
super.onNewToken(token);
Airbridge.registerPushToken(token);
}
}
class MyFirebaseMessagingService : FirebaseMessagingService() {
override fun onMessageReceived(remoteMessage: RemoteMessage) {
super.onMessageReceived(remoteMessage)
}
override fun onNewToken(token: String) {
super.onNewToken(token)
Airbridge.registerPushToken(token)
}
}
A push token may already have been issued by the FCM service.
Send this token as well through the Airbridge SDK as below.
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
FirebaseMessaging.getInstance()
.getToken()
.addOnSuccessListener(new OnSuccessListener<String>() {
@Override
public void onSuccess(String token) {
Airbridge.registerPushToken(token);
}
});
}
}
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
...
FirebaseMessaging.getInstance()
.token
.addOnSuccessListener {
Airbridge.registerPushToken(it)
}
...
}
}
Please make sure that the above functions are called after the SDK initialization process.
Silent notifications are sent without any data. However, the notification may be shown to the user depending on the onMessageReceived
settings.
Please make sure to add an exception handling logic to prevent the display of silent notifications for users.
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
if (remoteMessage.getData().containsKey("airbridge-uninstall-tracking")) {
return;
} else {
// handleNotification(remoteMessage);
}
}
@Override
public void onNewToken(String token) {
super.onNewToken(token);
Airbridge.registerPushToken(token);
}
}
class MyFirebaseMessagingService : FirebaseMessagingService() {
override fun onMessageReceived(remoteMessage: RemoteMessage) {
super.onMessageReceived(remoteMessage)
if (remoteMessage.data.containsKey("airbridge-uninstall-tracking")) {
return
} else {
// handleNotification(remoteMessage)
}
}
override fun onNewToken(token: String) {
super.onNewToken(token)
Airbridge.registerPushToken(token)
}
}
Troubleshooting
Morpheus Push SDK Troubleshooting
Updated almost 2 years ago